Unityにパッケージをインストール
Releases · googleads/googleads-mobile-unity
OfficialUnityPluginfortheGoogleMobileAdsSDK-googleads/googleads-mobile-unity
こちらの最新版をDLしてインポートする
広告設定
Assets/GoogleMobileAds/Resources/GoogleMobileAdsSettings.asset
上記パスに設定ファイルがあるため、そちらにAdMobで登録したAppIDを記載する
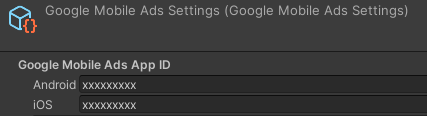
バナー広告設定
設定ファイルモデルを作成して設定を追加していく
以下のCSファイルを作成すると、CreateAssetMenuから設定アセットを作成できる。
作成した設定ファイルはResourcesフォルダに追加する。
using GoogleMobileAds.Api;
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
[CreateAssetMenu()]
public class AdMobSetting : ScriptableObject
{
#region バナー広告設定
[Header("バナー広告の設定")]
// バナー広告のID
public string bannerAdUnitId;
// バナー広告のサイズ
public enum BannerSizeType
{
Banner, // 320x50
MediumRectangle, // 300x250
Leaderboard, // 728x90
IABBanner, // 468x60
}
public BannerSizeType bannerSizeType = BannerSizeType.Banner;
public AdSize GetBannerSize()
{
switch (bannerSizeType)
{
case BannerSizeType.Banner:
return AdSize.Banner;
case BannerSizeType.MediumRectangle:
return AdSize.MediumRectangle;
case BannerSizeType.Leaderboard:
return AdSize.Leaderboard;
case BannerSizeType.IABBanner:
return AdSize.IABBanner;
default:
return AdSize.Banner;
}
}
// バナー広告の表示位置
public AdPosition bannerAdPosition = AdPosition.Bottom;
#endregion
}
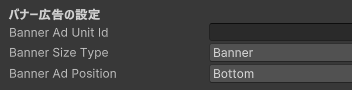
上記画像のようにバーナーの設定を行えるようになる。
バナー広告の実装
以下のCSファイルを作成する。
LoadAd()を呼び出してロードが完了すると自動でAdが表示される。
使い方としては表示するときに new AdMobBannerAd(); するだけである。
using GoogleMobileAds.Api;
using System;
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class AdMobBannerAd : IDisposable
{
private BannerView bannerView;
public AdMobBannerAd()
{
var setting = AdMobManager.GetSetting();
var bannerId = setting.bannerAdUnitId;
var bannerSize = setting.GetBannerSize();
var position = setting.bannerAdPosition;
bannerView = new BannerView(bannerId, bannerSize, position);
ListenToAdEvents();
LoadAd();
}
public void LoadAd()
{
var adRequest = new AdRequest();
bannerView.LoadAd(adRequest);
}
private void ListenToAdEvents()
{
// バナー広告の読み込みが完了したとき
bannerView.OnBannerAdLoaded += () =>
{
Debug.Log("Banner ad loaded");
};
// バナー広告の読み込みに失敗したとき
bannerView.OnBannerAdLoadFailed += (LoadAdError error) =>
{
Debug.LogError(error);
};
// バナー広告がクリックされたとき
bannerView.OnAdClicked += () =>
{
Debug.Log("Banner ad clicked");
};
}
private void DestroyAd()
{
if (bannerView != null)
{
bannerView.Destroy();
bannerView = null;
}
}
public void Dispose()
{
DestroyAd();
}
}
AdMob管理クラス
AdMobを管理する用のクラス
使い方としては AdMobManager.Initialize(); を呼ぶだけでAdMobの機能が使えるようになる。
using GoogleMobileAds.Api;
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public static class AdMobManager
{
private const string AdMobSettingPath = "AdMobSetting";
private static AdMobBannerAd bannerAd;
private static AdMobSetting setting;
public static AdMobSetting GetSetting() => setting;
public static bool IsInitialized { get; private set; }
public static void Initialize()
{
LoadSetting();
MobileAds.Initialize(initStatus =>
{
Debug.Log(initStatus);
});
IsInitialized = true;
}
private static void LoadSetting()
{
if (setting == null)
{
setting = Resources.Load<AdMobSetting>(AdMobSettingPath);
}
}
public static void ShowBannerAd()
{
if (bannerAd == null)
{
bannerAd = new AdMobBannerAd();
}
}
public static void HideBannerAd()
{
if (bannerAd != null)
{
bannerAd.Dispose();
}
}
public static void Shutdown()
{
if (bannerAd != null)
{
bannerAd.Dispose();
}
}
}
動作
設定ファイルに合わせてバナー広告が表示される
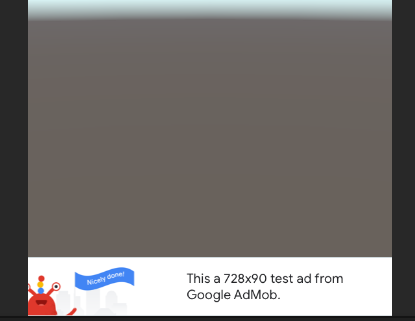
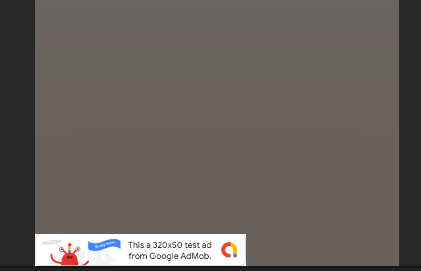
トラブルシューティング
以下のエラーが出るとき、Unity側のバグなのでEditorのバージョンを上げる必要がある。
ArgumentNullException: Value cannot be null.
Parameter name: _unity_self
ドキュメント
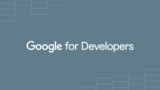
始める | Unity | Google for Developers
Unityでアプリを開発しているAdMobパブリッシャー向けのモバイル広告用SDKです。