よくある汎用的な表示方法をまとめています。(随時更新)
ボーダーライン(borderline)

Widget widgetExample() {
return Padding(
padding: const EdgeInsets.only(left: 20, right: 20, top: 8, bottom: 8),
child: Container(
height: 2,
decoration: const BoxDecoration(
color: Colors.black87,
borderRadius: BorderRadius.all(Radius.circular(4.0)),
),
),
);
}
ゲージ(gauge)
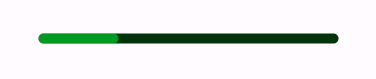
Widget widgetExample() {
return Padding(
padding: const EdgeInsets.only(top: 4),
child: Container(
height: 10,
width: 300,
// ゲージ背景
decoration: const BoxDecoration(
color: Color.fromARGB(255, 2, 51, 10),
borderRadius: BorderRadius.all(Radius.circular(64)),
),
// ゲージ部分
child: Row(
children: [
Container(
height: 10,
width: 80,
decoration: BoxDecoration(
color: const Color.fromARGB(255, 0, 255, 55).withOpacity(0.5),
borderRadius: const BorderRadius.all(Radius.circular(64)),
),
),
],
),
),
);
}
複数のトグルボタン(ToggleButtons)

enum Category { book, video, game }
...
Category currentCategory = Category.book;
// 3つのボタンを並べる
Widget widgetExample() {
return Padding(
padding: const EdgeInsets.only(left: 16, right: 16),
child: Container(
height: 50,
child: Row(
children: <Widget>[
button(Category.book, currentCategory == Category.book),
const SizedBox(
width: 16,
),
button(Category.video, currentCategory == Category.video),
const SizedBox(
width: 16,
),
button(Category.game, currentCategory == Category.game),
],
),
),
);
}
// 1ボタン
Widget button(Category categoryType, bool isSelected) {
String txt = '';
if (Category.book == categoryType) {
txt = 'Ui/Ux';
} else if (Category.video == categoryType) {
txt = 'Coding';
} else if (Category.game == categoryType) {
txt = 'Basic UI';
}
return Expanded(
child: Container(
decoration: BoxDecoration(
color: isSelected ? Colors.blue : Colors.white70,
borderRadius: const BorderRadius.all(Radius.circular(24.0)),
border: Border.all(color: Colors.blue)),
child: Material(
color: Colors.transparent,
child: InkWell(
splashColor: Colors.white24,
borderRadius: const BorderRadius.all(Radius.circular(24.0)),
onTap: () {
setState(() {
currentCategory = categoryType;
});
},
child: Padding(
padding: const EdgeInsets.only(
top: 12, bottom: 12, left: 18, right: 18),
child: Center(
child: Text(
txt,
textAlign: TextAlign.left,
style: TextStyle(
fontWeight: FontWeight.w600,
fontSize: 16,
letterSpacing: 0.27,
color: isSelected ? Colors.white70 : Colors.blue,
),
),
),
),
),
),
),
);
}
Boxの中にデザインを入れて切り抜く(Rect Image)
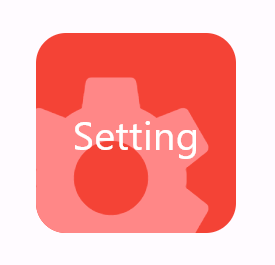
Widget widgetExample() {
return Container(
height: 200,
width: 200,
decoration: BoxDecoration(
borderRadius: BorderRadius.circular(30),
color: Colors.red,
),
// ChipRRectで角丸にしつつ、中のコンテンツを切り抜いている
child: const ClipRRect(
clipBehavior: Clip.antiAlias,
borderRadius: BorderRadius.all(Radius.circular(30)),
// Stackで要素を重ねている
child: Stack(
children: [
// Positionedで左下にギアのアイコンを配置
Positioned(
top: 20,
left: -50,
child: Icon(
Icons.settings,
size: 250,
color: Color.fromARGB(255, 255, 135, 135),
),
),
// Alingで中央にテキストを配置
Align(
alignment: Alignment.center,
child: Text(
"Setting",
style: TextStyle(color: Colors.white, fontSize: 40),
),
),
],
),
),
);
}
テキストボックス
通常のテキストボックス(Text Box)

String inputText = "";
Widget widgetExample() {
return TextField(
autofocus: true, // 自動で選択状態にする
decoration: const InputDecoration(
hintText: "ヒントテキスト", // 入力していない時のテキスト
// border: InputBorder.none // 下線を消す
),
onChanged: (value) => setState(() {
inputText = value;
}),
);
}
金額用のテキストボックス(Price Text Box)

パッケージ追加
テキストを通過に変換してくれるパッケージ
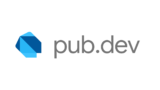
currency_text_input_formatter | Flutter package
CurrencyTextInputFormatterforFlutter.Useiteasyandsimpleforyourflutterapp.
currency_text_input_formatter: ^2.1.10
処理
String inputText = "";
final String symbol = '¥';
final TextEditingController _controller =
TextEditingController(); // システム側でテキストを書き換えるためのコントローラー
Widget widgetExample() {
return TextField(
controller: _controller,
// ヒントテキスト
decoration: InputDecoration(
hintText: "${symbol}0",
),
// 数字用のキーボードを表示する
keyboardType: TextInputType.number,
// 入力されたものを自動でで変換する
inputFormatters: [
CurrencyTextInputFormatter(
decimalDigits: 1, // 少数点以下の桁数
symbol: symbol, // 通貨シンボル
)
],
onChanged: (value) => setState(() {
inputText = value;
// すべての入力が消された時、0を表示する
if (value == "") {
_controller.text = "${symbol}0";
}
}),
);
}
キーボード出現時に自動で動かないようにする
Scaffold(
// falseで自動で動かないようになる
resizeToAvoidBottomInset: false,
)
iOSシミュレーターでキーボードが出ない時
iOSのシミュレータのメニューバーから
[ I/O ] -> [ keyboard ] -> [ connectHardwareKeybard ]
を OFF にすることで表示されるようになる